Class Job
Represents a job.
[Serializable]
[JsonObject(MemberSerialization.OptIn)]
public class Job : CustomDataType, DataType, IManagerIdentifiableObject<JobID>, ITrackLastModified, ISectionContainer
- Inheritance
-
Job
- Implements
-
IManagerIdentifiableObject<JobID>
- Extension Methods
Examples
JobManagerHelper jobManagerHelper = new JobManagerHelper(msg => protocol.SLNet.RawConnection.HandleMessages(msg));
var sectionDefinition = jobManagerHelper.SectionDefinitions.Read(SectionDefinitionExposers.Name.Equal("MyCustomSectionDefinition")).FirstOrDefault() as CustomSectionDefinition;
var fieldDescriptor = sectionDefinition.GetAllFieldDescriptors().FirstOrDefault(x => x.Name == "MyStringField");
var startTime = DateTime.UtcNow;
var endTime = startTime.AddHours(10);
Job job = new Job();
job.SetJobName("MyJob");
job.SetJobStartTime(startTime);
job.SetJobEndTime(endTime);
// Add a section to the Job.
var section = new Section(sectionDefinition);
section.AddOrUpdateValue(fieldDescriptor, "My field value.");
job.Sections.Add(section);
jobManagerHelper.Jobs.Create(job);
Remarks
A job consists of a number of sections and each section in turn consists of a number of FieldValues.
A job may contain multiple sections with the same SectionDefinition.
The following diagram gives an overview of the different classes in the Jobs API:
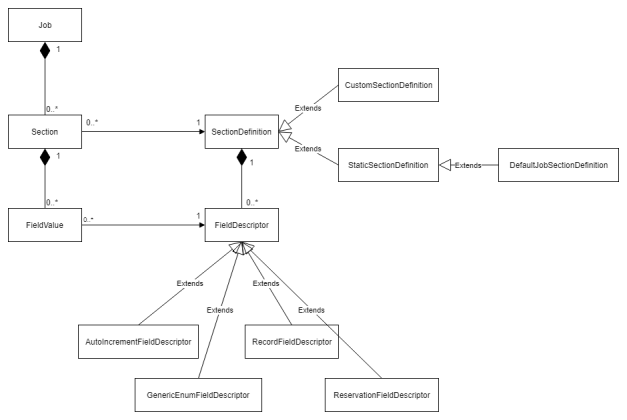
Constructors
- Job(SerializationInfo, StreamingContext)
Initializes a new instance of the Job class using the specified serialization info and streaming context.
Properties
- ID
Gets or sets the job ID.
- JobDomainID
Gets or sets the Job domain ID.
- Sections
Gets the sections of this job.
- SecurityViewID
Gets or sets the security view ID. Available from DataMiner 10.0.5 (RN 24800) onwards.
- SecurityViewIDs
Gets or sets the security view IDs. Available from DataMiner 10.1.1 (RN 28311) onwards.
- WasStitched
Gets a value indicating whether this job was stitched.
Methods
- AsJsonSerializableDictionary()
Returns this job as a JSON serializable dictionary.
- Clone()
Creates a new object that is a copy of the current instance.
- Equals(Job)
Indicates whether the current object is equal to another object of the same type.
- Equals(object)
Determines whether the specified object is equal to the current object.
- FromJson(string)
Deserializes the specified JSON string into a new instance of this class.
- GetHashCode()
Calculates the hash code for this object.
- GetJobDomain()
Returns the JobDomain when the Job has been stitched
- GetObjectData(SerializationInfo, StreamingContext)
Populates a SerializationInfo with the data needed to serialize the target object.
- GetSecurityViewIds()
Gets the security view IDs.
- Stitch(Func<SectionDefinitionID, SectionDefinition>)
Stitches this job with the specified section definition provider.
- Stitch(Func<SectionDefinitionID, SectionDefinition>, Func<JobDomainID, JobDomain>)
Stitches this job with the specified section definition provider and job domain provider.
- ToFilter<T>()
Converts this job to an ID-based filter of the specified type.
- ToJson()
Retrieves the JSON representation of this object.
- ToString()
Returns a string that represents the current object.